For those of you who develop JIL widgets but do not want to use the SDK provided by JIL (or who cannot use it because it is not available for your software platform), there is now a way to have code completion for JIL APIs in any modern IDE. I created a JavaScript file that models all JIL APIs found in v1.2.2 of the JIL Handset API specification. Just include it into your widget project and if your IDE supports code completion for objects defined in other files, you will have code completion for the JIL APIs.
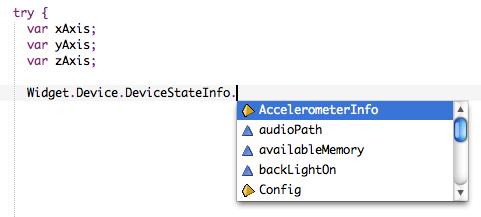
This file will not emulate any functionality, it just describes the JIL APIs to make it easier to code your widget in other IDEs than the editor included in the JIL SDK. You should make sure that you do not include the file once you deploy your widget onto a device, because it could interfere with the “real” Widget-object provided by the widget runtime.
What I would like to do for future versions is to include the description found in the specification into the file to have documentation for the APIs as well, but I am not sure if I am allowed to do that. I have to get confirmation from JIL first.
/**
* Code completion for JIL Widgets<br />
* <br />
* Based upon JIL Widget System High Level Technical Specification -
* Handset APIs v1.2.2<br />
* <br />
* This file does not emulate any of the JIL APIs, it just describes the
* JIL APIs so that a developer, by including this file into his widget project,
* gets code completion for the JIL APIs outside of the JIL SDK.<br />
* <br />
*
* @author Stefan Kolb stefan.kolb@indiginox.com
* @version 0.1.0
*/
/**
* Central JIL namespace object<br />
* <br />
* Feature URI: http://jil.org/jil/api/1.1/widget
*
* @namespace Widget
* @version 1.2.2
*/
var Widget = {
/**
* @event
* @type void
*/
onFocus: function() { },
/**
* @event
* @type void
*/
onMaximize: function() { },
/**
* @event
* @type void
*/
onRestore: function() { },
/**
* @event
* @type void
*/
onWakeup: function() { },
/**
* @param {string} url
* @type void
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
*/
openURL: function(url) { },
/**
* @param {string} key
* @type string
* @throws {{Widget.ExceptionTypes.INVALID_PARAMETER}}
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
*/
preferenceForKey: function(key) { },
/**
* @param {string} preference
* @param {string} key
* @type void
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
* @throws {{Widget.ExceptionTypes.INVALID_PARAMETER}}
*/
setPreferenceForKey: function(preferenceForKey, key) { }
};
/**
* Feature URI: http://jil.org/jil/api/1.1/device
*
* @namespace Widget.Device
* @version 1.2.2
*/
Widget.Device = {
/** @type string */
clipboardString: "",
/** @type string */
widgetEngineName: "",
/** @type string */
widgetEngineProvider: "",
/** @type string */
widgetEngineVersion: "",
/**
* @param {string} originalFile
* @param {string} destinationFullName
* type boolean
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
* @throws {Widget.ExceptionTypes.SECURITY}
* @throws {Widget.ExceptionTypes.INVALID_PARAMETER}
*/
copyFile: function(originalFile, destinationFullName) { },
/**
* @param {string} destinationFullName
* @type boolean
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
* @throws {Widget.ExceptionTypes.INVALID_PARAMETER}
* @throws {Widget.ExceptionTypes.SECURITY}
*/
deleteFile: function(destinationFullName) { },
/**
* @param {File} matchFile
* @param {Number} startInx
* @param {Number} endInx
* @type void
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
* @throws {Widget.ExceptionTypes.INVALID_PARAMETER}
*/
findFiles: function(matchFile, startInx, endInx) { },
/**
* @type array
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
* @throws {Widget.ExceptionTypes.SECURITY}
*/
getAvailableApplications: function() { },
/**
* @param {string} sourceDirectory
* @type array
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
* @throws {Widget.ExceptionTypes.SECURITY}
* @throws {Widget.ExceptionTypes.INVALID_PARAMETER}
*/
getDirectoryFileNames: function(sourceDirectory) { },
/**
* @param {string} fullName
* @type File
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
* @throws {Widget.ExceptionTypes.SECURITY}
* @throws {Widget.ExceptionTypes.INVALID_PARAMETER}
*/
getFile: function(fullName) { },
/**
* @type array
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
* @throws {Widget.ExceptionTypes.SECURITY}
*/
getFileSystemRoots: function() { },
/**
* @param {string} fileSystemRoot
* @type Number
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
* @throws {Widget.ExceptionTypes.INVALID_PARAMETER}
*/
getFileSystemSize: function(fileSystemRoot) { },
/**
* @param {string} application
* @param {string} startParemeter
* @type void
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
* @throws {Widget.ExceptionTypes.SECURITY}
* @throws {Widget.ExceptionTypes.INVALID_PARAMETER}
*/
launchApplication: function(application, startParameter) { },
/**
* @param {string} originalFile
* @param {string} destinationFullName
* @type boolean
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
* @throws {Widget.ExceptionTypes.INVALID_PARAMETER}
* @throws {Widget.ExceptionTypes.SECURITY}
*/
moveFile: function(originalFile, destionationFullName) { },
/**
* @event
* @param {array} filesFound
* @type void
*/
onFilesFound: function(filesFound) { },
/**
* @param {string} ringtoneFileurl
* @param {AddressBookItem} addressBookItem
* @type void
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
* @throws {Widget.ExceptionTypes.SECURITY}
* @throws {Widget.ExceptionTypes.INVALID_PARAMETER}
*/
setRingtone: function(ringtoneFileurl, addressBookItem) { },
/**
* @param {Number} durationSeconds
* @type void
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
* @throws {Widget.ExceptionTypes.INVALID_PARAMETER}
*/
vibrate: function(durationSeconds) { }
};
/**
* Feature URI: http://jil.org/jil/api/1.1/accountinfo
*
* @namespace Widget.Device.AccountInfo
* @version 1.2.2
*/
Widget.Device.AccountInfo = {
/** @type string */
phoneMSISDN: "",
/** @type string */
phoneOperatorName: "",
/** @type string */
phoneUserUniqueId: "",
/** @type string */
userAccountBalance: "",
/** @type string */
userSubscriptionType: ""
};
/**
* Feature: http://jil.org/jil/api/1.1.5/applicationtypes
*
* @namespace Widget.Device.ApplicationTypes
* @version 1.2.2
*/
Widget.Device.ApplicationTypes = {
/** @type string */
ALARM: "",
/** @type string */
BROWSER: "",
/** @type string */
CALCULATOR: "",
/** @type string */
CALENDAR: "",
/** @type string */
CAMERA: "",
/** @type string */
CONTACTS: "",
/** @type string */
FILES: "",
/** @type string */
GAMES: "",
/** @type string */
MAIL: "",
/** @type string */
MEDIAPLAYER: "",
/** @type string */
MESSAGING: "",
/** @type string */
PHONECALL: "",
/** @type string */
PICTURES: "",
/** @type string */
PROG_MANAGER: "",
/** @type string */
SETTINGS: "",
/** @type string */
TASKS: "",
/** @type string */
WIDGET_MANAGER: ""
};
/**
* Feature URI: http://jil.org/jil/api/1.1/deviceinfo
*
* @namespace Widget.Device.DeviceInfo
* @version 1.2.2
*/
Widget.Device.DeviceInfo = {
/** @type Widget.PIM.AddressBookItem */
ownerInfo: { },
/** @type number */
phoneColorDepthDefault: new Number(),
/** @type string */
phoneFirmware: "",
/** @type string */
phoneManufacturer: "",
/** @type string */
phoneModel: "",
/** @type string */
phoneOS: "",
/** @type number */
phoneScreenHeightDefault: new Number(),
/** @type number */
phoneScreenWidthDefault: new Number(),
/** @type string */
phoneSoftware: "",
/** @type number */
totalMemory: ""
};
/**
* Feature URI: http://jil.org/jil/api/1.1.1/datanetworkinfo
*
* @namespace Widget.Device.DataNetworkInfo
* @version 1.2.2
*/
Widget.Device.DataNetworkInfo = {
/** @type boolean */
isDataNetworkConnected: new Boolean(),
/** @type array */
networkConnectionType: [ ],
/**
* @param {string} networkConnectionType
* @type string
* @throws {Widget.ExeceptionTypes.UNSUPPORTED}
* @throws {Widget.ExeceptionTypes.INVALID_PARAMETER}
*/
getNetworkConnectionName: function(networkConnectionType) { },
/**
* @event
* @param {string} newConnectionName
* @type void
*/
onNetworkConnectionChanged: function(newConnectionName) { }
};
/**
* Feature URI: http://jil.org/jil/api/1.1.5/datanetworkconnectiontypes
*
* @namespace Widget.DeviceDataNetworkInfo.DataNetworkConnectionTypes
* @version 1.2.2
*/
Widget.Device.DataNetworkInfo.DataNetworkConnectionTypes = {
/** @type string */
BLUETOOTH: "",
/** @type string */
EDGE: "",
/** @type string */
EDVO: "",
/** @type string */
GPRS: "",
/** @type string */
IRDA: "",
/** @type string */
LTE: "",
/** @type string */
ONEXRTT: "",
/** @type string */
WIFI: ""
};
/**
* Feature URI: http://jil.org/jil/api/1.1/devicestateinfo
*
* @namespace Widget.Device.DeviceStateInfo
* @version 1.2.2
*/
Widget.Device.DeviceStateInfo = {
/** @type string */
audioPath: "",
/** @type number */
availableMemory: new Number(),
/** @type boolean */
backLightOn: new Boolean(),
/** @type boolean */
keypadLightOn: new Boolean(),
/** @type string */
language : "",
/** @type string */
processorUtilizationPercent: "",
/**
* @event
* @param {boolean} isNowClosed
* @type void
*/
onFlipEvent: function(isNowClosed) { },
/**
* @event
* @param {PositionInfo} locationinfo
* @param {string} method
* @type void
*/
onPositionRetrieved: function(locationinfo, method) { },
/**
* @event
* @param {number} newWidth
* @param {number} newHeight
* @type void
*/
onScreenChangeDimensions: function(newWidth, newHeight) { },
/**
* @param {string} method
* @type void
* @throws {Widget.ExceptionTypes.UNSUPPORTED},
* @throws {Widget.ExceptionTypes.SECURITY},
* @throws {Widget.ExceptionTypes.INVALID_PARAMETER}
*/
requestPositionInfo: function(method) { }
};
/**
* Feature URI: http://jil.org/jil/api/1.1/accelerometerinfo
*
* @namespace Widget.Device.DeviceStateInfo.AccelerometerInfo
* @version 1.2.2
*/
Widget.Device.DeviceStateInfo.AccelerometerInfo = {
/** @type number */
xAxis: new Number(),
/** @type number */
yAxis: new Number(),
/** @type number */
zAxis: new Number()
};
/**
* Feature URI: http://jil.org/jil/api/1.1/config
*
* @namespace Widget.Device.DeviceStateInfo.Config
* @version 1.2.2
*/
Widget.Device.DeviceStateInfo.Config = {
/** @type number */
msgRingtoneVolume: new Number(),
/** @type number */
ringtoneVolume: new Number(),
/** @type string */
vibrationSetting: "",
/**
* @param {string} wallpaperFileurl
* @type void
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
* @throws {Widget.ExceptionTypes.SECURITY}
* @throws {Widget.ExceptionTypes.INVALID_PARAMETER}
*/
setAsWallpaper: function(wallpaperFileurl) { },
/**
* @param {string} ringtoneFileurl
* @type void
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
* @throws {Widget.ExceptionTypes.SECURITY}
* @throws {Widget.ExceptionTypes.INVALID_PARAMETER}
*/
setDefaultRingtone: function(ringtoneFileurl) { }
};
/**
* Feature URI: http://jil.org/jil/api/1.1.1/file
*
* @namespace Widget.Device.File
* @version 1.2.2
*/
Widget.Device.File = {
/** @type date */
createDate: new Date(),
/** @type string */
fileName: "",
/** @type string */
filePath: "",
/** @type number */
fileSize: new Number(),
/** @type boolean */
isDirectory: new Boolean(),
/** @type date */
lastModifyDate: new Date()
};
/**
* Feature URI: http://jil.org/jil/api/1.1/positioninfo
*
* @namespace Widget.Device.PositionInfo
* @version 1.2.2
*/
Widget.Device.PositionInfo = {
/** @type number */
accuracy: new Number(),
/** @type number */
altitude: new Number(),
/** @type number */
altitudeAccuracy: new Number(),
/** @type number */
cellID: new Number(),
/** @type number */
latitude: new Number(),
/** @type number */
longitude: new Number(),
/** @type Date */
timestamp: new Date()
};
/**
* Feature URI: http://jil.org/jil/api/1.1/powerinfo
*
* @namespace Widget.Device.PowerInfo
* @version 1.2.2
*/
Widget.Device.PowerInfo = {
/** @type boolean */
isCharging: new Boolean(),
/** @type number */
percentRemaining: new Number(),
/**
* @event
* @param {number} newPercentageRemaining
* @type void
*/
onChargeLevelChange: function(newPercentageRemainig) { },
/**
* @event
* @param {string} state
* @type void
*/
onChargeStateChange: function(state) { },
/**
* @event
* @param {number} percentRemaining
* @type void
*/
onLowBattery: function(precentRemaining) { }
};
/**
* Feature URI: http://jil.org/jil/api/1.1.1/radioinfo
*
* @namespace Widget.Device.RadioInfo
* @version 1.2.2
*/
Widget.Device.RadioInfo = {
/** @type boolean */
isRadioEnabled: new Boolean(),
/** @type boolean */
isRoaming: new Boolean(),
/** @type string */
radioSignalSource: "",
/** @type number */
radioSignalStrengthPercent: new Number(),
/**
* @event
* @param {string} signalSource
* @param {boolean} isRoaming
* @type void
*/
onSignalSourceChange: function(signalSource, isRoaming) { }
};
/**
* Feature URI: http://jil.org/jil/api/1.1.5/radiosignalsourcetypes
*
* @namespace Widget.Device.RadioInfo.RadioSignalSourceTypes
* @version 1.2.2
*/
Widget.Device.RadioInfo.RadioSignalSourceTypes = {
/** @type string */
CDMA: "",
/** @type string */
GSM: "",
/** @type string */
LTE: "",
/** @type string */
TDSCDMA: "",
/** @type string */
WCDMA: ""
};
/**
* @namespace Widget.Exception
*/
Widget.Exception = {
/** @type string */
message: "",
/** @type string */
type: ""
};
/**
* @namespace Widget.ExceptionTypes
*/
Widget.ExceptionTypes = {
/** @type string */
UNSUPPORTED: 'unsupported',
/** @type string */
SECURITY: 'security',
/** @type string */
INVALID_PARAMETER: 'invalid_parameter',
/** @type string */
UNKNOWN: 'unknown'
};
/**
* Feature URI: http://jil.org/jil/api/1.1/messaging
*
* @namespace Widget.Messaging
* @version 1.2.2
*/
Widget.Messaging = {
/**
* @param {Message} msg
* @param {string} destinationFolder
* @type void
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
* @throws {Widget.ExceptionTypes.SECURITY}
* @throws {Widget.ExceptionTypes.INVALID_PARAMETER}
*/
copyMessageToFolder: function(msg, destinationFolder) { },
/**
* @param {string} messageType
* @param {string} folderName
* @type void
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
* @throws {Widget.ExceptionTypes.SECURITY}
* @throws {Widget.ExceptionTypes.INVALID_PARAMETER}
*/
createFolder: function(messageType, folderName) { },
/**
* @param {string} messageType
* @type Message
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
* @throws {Widget.ExceptionTypes.SECURITY}
* @throws {Widget.ExceptionTypes.INVALID_PARAMETER}
*/
createMessage: function(messageType) { },
/**
* @param {string} messageType
* @param {string} folderName
* @type void
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
* @throws {Widget.ExceptionTypes.SECURITY}
* @throws {Widget.ExceptionTypes.INVALID_PARAMETER}
*/
deleteAllMessages: function(messageType, folderName) { },
/**
* @param {string} accountId
* @type void
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
* @throws {Widget.ExceptionTypes.SECURITY}
* @throws {Widget.ExceptionTypes.INVALID_PARAMETER}
*/
deleteEmailAccount: function(accountId) { },
/**
* @param {string} messageType
* @param {string} folderName
* @type void
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
* @throws {Widget.ExceptionTypes.SECURITY}
* @throws {Widget.ExceptionTypes.INVALID_PARAMETER}
*/
deleteFolder: function(messageType, folderName) { },
/**
* @param {string} messageType
* @param {string} folderName
* @param {string} id
* @type void
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
* @throws {Widget.ExceptionTypes.SECURITY}
* @throws {Widget.ExceptionTypes.INVALID_PARAMETER}
*/
deleteMessage: function(messageType, folderName, id) { },
/**
* @param {Message} comparisonMsg
* @param {string} folderName
* @param {number} startInx
* @param {number} endInx
* @type void
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
* @throws {Widget.ExceptionTypes.SECURITY}
* @throws {Widget.ExceptionTypes.INVALID_PARAMETER}
*/
findMessages: function(comparisonMsg, folderName, startInx, endInx) { },
/**
* @type Account
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
* @throws {Widget.ExceptionTypes.SECURITY}
*/
getCurrentEmailAccount: function() { },
/**
* @type array
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
* @throws {Widget.ExceptionTypes.SECURITY}
*/
getEmailAccounts: function() { },
/**
* @param {string} messageTypes
* @type array
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
* @throws {Widget.ExceptionTypes.SECURITY}
* @throws {Widget.ExceptionTypes.INVALID_PARAMETER}
*/
getFolderNames: function(messageTypes) { },
/**
* @param {string} messageType
* @param {string} folderName
* @param {number} index
* @type Message
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
* @throws {Widget.ExceptionTypes.SECURITY}
* @throws {Widget.ExceptionTypes.INVALID_PARAMETER}
*/
getMessage: function(messageType, folderName, index) { },
/**
* @param {string} messageType
* @param {string} folderName
* @type MessageQuantities
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
* @throws {Widget.ExceptionTypes.SECURITY}
* @throws {Widget.ExceptionTypes.INVALID_PARAMETER}
*/
getMessageQuantities: function(messageType, folderName) { },
/**
* @param {Message} msg,
* @param {string} destinationFolder
* @type void
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
* @throws {Widget.ExceptionTypes.SECURITY}
* @throws {Widget.ExceptionTypes.INVALID_PARAMETER}
*/
moveMessageToFolder: function(msg, destinationFolder) { },
/**
* @event
* @param {Message} msg
* @type void
*/
onMessageArrived: function(msg) { },
/**
* @event
* @param {Message} msg
* @param {string} error
* @type void
*/
onMessageSendingFailure: function(msg, error) { },
/**
* @event
* @param {array} messagesFound
* @param {string} folderName
* @type void
*/
onMessagesFound: function(messagesFound, folderName) { },
/**
* @param {Message} msg
* @type void
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
* @throws {Widget.ExceptionTypes.SECURITY}
* @throws {Widget.ExceptionTypes.INVALID_PARAMETER}
* @throws {Widget.ExceptionTypes.UNKNOWN}
*/
sendMessage: function(msg) { },
/**
* @param {string} accountId
* @type void
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
* @throws {Widget.ExceptionTypes.SECURITY}
* @throws {Widget.ExceptionTypes.INVALID_PARAMETER}
*/
setCurrentEmailAccount: function(accountId) { }
};
/**
* Feature URI: http://jil.org/jil/api/1.1/account
*
* @namespace Widget.Messaging.Account
* @version 1.2.2
*/
Widget.Messaging.Account = {
/** @type string */
accountId: "",
/** @type string */
accountName: ""
};
/**
* Feature URI: http://jil.org/jil/api/1.1/attachment
*
* @namespace Widget.Messaging.Attachment
* @version 1.2.2
*/
Widget.Messaging.Attachment = {
/** @type string */
fileName: "",
/** @type string */
MIMEType: "",
/** @type number */
size: new Number()
};
/**
* Feature URI: http://jil.org/jil/api/1.1/message
*
* @namespace Widget.Messaging.Message
* @version 1.2.2
*/
Widget.Messaging.Message = {
/** @type array */
attachments: [ ],
/** @type array */
bccAddress: [ ],
/** @type string */
body: "",
/** @type string */
callbackNumber: "",
/** @type array */
ccAddress: [ ],
/** @type array */
destinationAddress: [ ],
/** @type boolean */
isRead: new Boolean(),
/** @type string */
messageId: "",
/** @type boolean */
messagePriority: new Boolean(),
/** @type string */
messageType: "",
/** @type string */
sourceAddress: "",
/** @type string */
subject: "",
/** @type Date */
time: new Date(),
/** @type number */
validityPeriodHours: new Number(),
/**
* @param {string} type
* @param {string} address
* @type void
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
* @throws {Widget.ExceptionTypes.INVALID_PARAMETER}
*/
addAddress: function(type, address) { },
/**
* @param {string} fileFullName
* @type void
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
* @throws {Widget.ExceptionTypes.INVALID_PARAMETER}
*/
addAttachment: function(fileFullName) { },
/**
* @param {string} type
* @param {string} address
* @type void
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
* @throws {Widget.ExceptionTypes.SECURITY}
* @throws {Widget.ExceptionTypes.INVALID_PARAMETER}
*/
deleteAddress: function(type, address) { },
/**
* @param {Attachment} attachment
* @type void
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
* @throws {Widget.ExceptionTypes.SECURITY}
* @throws {Widget.ExceptionTypes.INVALID_PARAMETER}
*/
deleteAttachment: function(attachment) { },
/**
* @param {string} fileFullName
* @param {Attachment} attachment
* @type void
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
* @throws {Widget.ExceptionTypes.SECURITY}
* @throws {Widget.ExceptionTypes.INVALID_PARAMETER}
*/
saveAttachment: function(fileFullName, attachment) { }
};
/**
* Feature URI: http://jil.org/jil/api/1.1.4/messagefoldertypes
*
* @namespace Widget.Messaging.MessageFolderTypes
* @version 1.2.2
*/
Widget.Messaging.MessageFolderTypes = {
/** @type string */
DRAFTS: "",
/** @type string */
INBOX: "",
/** @type string */
OUTBOX: "",
/** @type string */
SENTBOX: ""
};
/**
* Feature URI: http://jil.org/jil/api/1.1/messagequantities
*
* @namespace Widget.Messaging.MessageQuantities
* @version 1.2.2
*/
Widget.Messaging.MessageQuantities = {
/** @type number */
totalMessageCnt: new Number(),
/** @type number */
totalMessageReadCnt: new Number(),
/** @type number */
totalMessageUnreadCnt: new Number()
};
/**
* Feature URI: http://jil.org/jil/api/1.1/messagetypes
*
* @namespace Widget.Message.MessageTypes
* @version 1.2.2
*/
Widget.Messaging.MessageTypes = {
/** @type string */
EmailMessage: "",
/** @type string */
MMSMessage: "",
/** @type string */
SMSMessage: ""
};
/**
* Feature URI: http://jil.org/jil/api/1.1/multimedia
*
* @namespace Widget.Multimedia
* @version 1.2.2
*/
Widget.Multimedia = {
/** @type boolean */
isAudioPlaying: new Boolean(),
/** @type boolean */
isVideoPlaying: new Boolean(),
/**
* @type void
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
*/
getVolume: function() { },
/**
* @type void
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
*/
stopAll: function() { }
};
/**
* Feature URI: http://jil.org/jil/api/1.1/audioplayer
*
* @namespace Widget.Multimedia.AudioPlayer
* @version 1.2.2
*/
Widget.Multimedia.AudioPlayer = {
/**
* @event
* @param {string} state
* @type void
*/
onStateChange: function(state) { },
/**
* @param {string} fileUrl
* @type void
*/
open: function(fileUrl) { },
/**
* @type void
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
*/
pause: function() { },
/**
* @param {number} repeatTimes
* @type void
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
* @throws {Widget.ExceptionTypes.INVALID_PARAMETER}
*/
play: function(repeatTimes) { },
/**
* @type void
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
*/
resume: function() { },
/**
* @type void
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
*/
stop: function() { }
};
/**
* Feature URI: http://jil.org/jil/api/1.1.2/camera
*
* @namespace Widget.Multimedia.Camera
* @version 1.2.2
*/
Widget.Multimedia.Camera = {
/**
* @param {string} fileName
* @param {string} lowRes
* @type string
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
* @throws {Widget.ExceptionTypes.SECURITY}
* @throws {Widget.ExceptionTypes.INVALID_PARAMETER}
*/
captureImage: function(fileName, lowRes) { },
/**
* @event
* @param {string} fileName
* @type void
*/
onCameraCaptured: function(fileName) { },
/**
* @param {object} domObj
* @type void
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
* @throws {Widget.ExceptionTypes.INVALID_PARAMETER}
*/
setWindow: function(domObj) { },
/**
* @param {string} fileName
* @param {boolean} lowRes
* @param {number} maxDurationSeconds
* @param {boolean} showDefaultControls
* @type string
* @@throws {Widget.ExceptionTypes.UNSUPPORTED}
* @throws {Widget.ExceptionTypes.SECURITY}
* @throws {Widget.ExceptionTypes.INVALID_PARAMETER}
*/
startVideoCapture: function(fileName, lowRes, maxDurationSeconds, showDefaultControls) { },
/**
* @type void
*/
stopVideoCapture: function() { }
};
/**
* Feature URI: http://jil.org/jil/api/1.1.2/videoplayer
*
* @namespace Widget.Multimedia.VideoPlayer
* @version 1.2.2
*/
Widget.Multimedia.VideoPlayer = {
/**
* @event
* @param {string} state
* @type void
*/
onStateChange: function(state) { },
/**
* @param {string} fileUrl
* @type void
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
* @throws {Widget.ExceptionTypes.INVALID_PARAMETER}
*/
open: function(fileUrl) { },
/**
* @type void
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
*/
pause: function() { },
/**
* @param {number} repeatTimes
* @type void
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
* @throws {Widget.ExceptionTypes.INVALID_PARAMETER}
*/
play: function(repeatTimes) { },
/**
* @type void
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
*/
resume: function() { },
/**
* @param {object} domObj
* @type void
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
* @throws {Widget.ExceptionTypes.INVALID_PARAMETER}
*/
setWindow: function(domObj) { },
/**
* @type void
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
*/
stop: function() { }
};
/**
* Feature URI: http://jil.org/jil/api/1.1.1/pim
*
* @namespace Widget.PIM
* @version 1.2.2
*/
Widget.PIM = {
/**
* @param {AddressBookItem} contact
* @type void
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
* @throws {Widget.ExceptionTypes.SECURITY}
* @throws {Widget.ExceptionTypes.INVALID_PARAMETER}
*/
addAddressBookItem: function(contact) { },
/**
* @param {CalendarItem} item
* @type void
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
* @throws {Widget.ExceptionTypes.SECURITY}
* @throws {Widget.ExceptionTypes.INVALID_PARAMETER}
*/
addCalendarItem: function(item) { },
/**
* @param {string} groupName
* @type void
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
* @throws {Widget.ExceptionTypes.SECURITY}
* @throws {Widget.ExceptionTypes.INVALID_PARAMETER}
*/
createAddressBookGroup: function(groupName) { },
/**
* @type AddressBookItem
* @throws Widget.ExceptionTypes.UNSUPPORED
* @throws {Widget.ExceptionTypes.SECURITY}
* @throws {Widget.ExceptionTypes.INVALID_PARAMETER}
*/
createAddressBookItem: function() { },
/**
* @param {string} groupName
* @type void
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
* @throws {Widget.ExceptionTypes.SECURITY}
* @throws {Widget.ExceptionTypes.INVALID_PARAMETER}
*/
deleteAddressBookGroup: function(groupName) { },
/**
* @param {string} id
* @type void
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
* @throws {Widget.ExceptionTypes.SECURITY}
* @throws {Widget.ExceptionTypes.INVALID_PARAMETER}
*/
deleteAddressBookItem: function(id) { },
/**
* @param {string} calendarId
* @type void
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
* @throws {Widget.ExceptionTypes.SECURITY}
* @throws {Widget.ExceptionTypes.INVALID_PARAMETER}
*/
deleteCalendarItem: function(calendarId) { },
/**
* @param {array} addressBookItems
* @void
*/
exportAsVCard: function(addressBookItems) { },
/**
* @param {AddressBookItem} comparisonContact
* @param {number} startInx
* @param {number} endInx
* @type void
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
* @throws {Widget.ExceptionTypes.SECURITY}
* @throws {Widget.ExceptionTypes.INVALID_PARAMETER}
*/
findAddressBookItems: function(comparisonContact, startInx, endInx) { },
/**
* @param {CalendarItem} itemToMatch
* @param {number} startInx
* @param {number} endInx
* @type void
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
* @throws {Widget.ExceptionTypes.SECURITY}
* @throws {Widget.ExceptionTypes.INVALID_PARAMETER}
*/
findCalendarItems: function(itemToMatch, startInx, endInx) { },
/**
* @param {string} groupName
* @type void
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
* @throws {Widget.ExceptionTypes.SECURITY}
* @throws {Widget.ExceptionTypes.INVALID_PARAMETER}
*/
getAddressBookGroupMembers: function(groupName) { },
/**
* @param {string} id
* @type AddressBookItem
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
* @throws {Widget.ExceptionTypes.SECURITY}
* @throws {Widget.ExceptionTypes.INVALID_PARAMETER}
*/
getAddressBookItem: function(id) { },
/**
* @type number
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
* @throws {Widget.ExceptionTypes.SECURITY}
* @throws {Widget.ExceptionTypes.INVALID_PARAMETER}
*/
getAddressBookItemsCount: function() { },
/**
* @type array
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
* @throws {Widget.ExceptionTypes.SECURITY}
*/
getAvailableAddressGroupNames: function() { },
/**
* @param {string} calendarId
* @type CalendarItem
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
* @throws {Widget.ExceptionTypes.SECURITY}
* @throws {Widget.ExceptionTypes.INVALID_PARAMETER}
*/
getCalendarItem: function(calendarId) { },
/**
* @param {Date} startTime
* @param {Date} endTime
* @type array
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
* @throws {Widget.ExceptionTypes.SECURITY}
* @throws {Widget.ExceptionTypes.INVALID_PARAMETER}
*/
getCalendarItems: function(startTime, endTime) { },
/**
* @event
* @param {array} addressBookItemsFound
* @type void
*/
onAddressBookItemsFound: function(addressBookItemsFound) { },
/**
* @event
* @param {CalendarItem} calendarItem
* @type void
*/
onCalendarItemAlert: function(calendarItem) { },
/**
* @param {array} calendarItemsFound
* @type void
*/
onCalendarItemsFound: function(calendarItemsFound) { },
/**
* @event
* @param {string} vCardFilePath
* @type void
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
* @throws {Widget.ExceptionTypes.INVALID_PARAMETER}
*/
onVCardExportingFinish: function(vCardFilePath) { }
};
/**
* Feature URI: http://jil.org/jil/api/1.1/addressbookitem
*
* @namespace Widget.PIM.AddressBookItem
* @version 1.2.2
*/
Widget.PIM.AddressBookItem = {
/** @type string */
address: "",
/** @type string */
addressBookItemId: "",
/** @type string */
company: "",
/** @type string */
eMail: "",
/** @type string */
fullName: "",
/** @type string */
homePhone: "",
/** @type string */
mobilePhone: "",
/** @type string */
title: "",
/** @type string */
workPhone: "",
/**
* @type array
*/
getAddressGroupNames: function() { },
/**
* @param {string} attribute
* @type string
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
* @throws {Widget.ExceptionTypes.INVALID_PARAMETER}
*/
getAttributeValue: function(attribute) { },
/**
* @type array
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
*/
getAvailableAttributes: function() { },
/**
* @param {array} groups
* @type void
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
* @throws {Widget.ExceptionTypes.INVALID_PARAMETER}
*/
setAddressGroupNames: function(groups) { },
/**
* @param {string} attribute
* @param {string} value
* @type void
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
* @throws {Widget.ExceptionTypes.INVALID_PARAMETER}
*/
setAttributeValue: function(attribute, value) { },
/**
* @type void
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
* @throws {Widget.ExceptionTypes.SECURITY}
* @throws {Widget.ExceptionTypes.INVALID_PARAMETER}
*/
update: function() { }
};
/**
* Feature URI: http://jil.org/jil/api/1.1/calendaritem
*
* @namespace Widget.PIM.CalendarItem
* @version 1.2.2
*/
Widget.PIM.CalendarItem = {
/** @type Date */
alarmDate: new Date(),
/** @type boolean */
alarmed: new Boolean(),
/** @type string */
calendarItemId: "",
/** @type Date */
eventEndTime: new Date(),
/** @type string */
eventName: "",
/** @type string */
eventNotes: "",
/** @type Date */
eventStartTime: new Date(),
/**
* @type void
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
* @throws {Widget.ExceptionTypes.SECURITY}
*/
update: function() { }
};
/**
* Feature URI: http://jil.org/jil/api/1.1/eventrecurrencetypes
*
* @namespace Widget.PIM.EventRecurrenceTypes
* @version 1.2.2
*/
Widget.PIM.EventRecurrenceTypes = {
/** @type string */
DAILY: "",
/** @type string */
EVERY_WEEKDAY: "",
/** @type string */
MONTHLY_ON_DAY: "",
/** @type string */
MONTHLY_ON_DAY_COUNT: "",
/** @type string */
NOT_REPEAT: "",
/** @type string */
WEEKLY_ON_DAY: "",
/** @type string */
YEARLY: ""
};
/**
* Feature URI: http://jil.org/jil/api/1.1.1/telephony
*
* @namespace Widget.Telephony
* @version 1.2.2
*/
Widget.Telephony = {
/**
* @param {string} callRecordType
* @type void
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
* @throws {Widget.ExceptionTypes.SECURITY}
* @throws {Widget.ExceptionTypes.INVALID_PARAMETER}
*/
deleteAllCallRecord: function(callRecordType) { },
/**
* @param {string} callRecordType
* @param {string} id
* @type void
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
* @throws {Widget.ExceptionTypes.SECURITY}
* @throws {Widget.ExceptionTypes.INVALID_PARAMETER}
*/
deleteCallRecord: function(callRecordType, id) { },
/**
* @param {CallRecord} comparisonRecord
* @param {number} startInx
* @param {number} endInx
* @type void
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
* @throws {Widget.ExceptionTypes.SECURITY}
* @throws {Widget.ExceptionTypes.INVALID_PARAMETER}
*/
findCallRecords: function(comparisonRecord, startInx, endInx) { },
/**
* @param {string} callRecordType
* @param {string} id
* @type CallRecord
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
* @throws {Widget.ExceptionTypes.SECURITY}
* @throws {Widget.ExceptionTypes.INVALID_PARAMETER}
*/
getCallRecord: function(callRecordType, id) { },
/**
* @param {string} callRecordType
* @type number
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
* @throws {Widget.ExceptionTypes.SECURITY}
* @throws {Widget.ExceptionTypes.INVALID_PARAMETER}
*/
getCallRecordCount: function(callRecordType) { },
/**
* @param {string} phoneNumber
* @type void
* @throws {Widget.ExceptionTypes.UNSUPPORTED}
* @throws {Widget.ExceptionTypes.SECURITY}
* @throws {Widget.ExceptionTypes.INVALID_PARAMETER}
*/
initiateVoiceCall: function(phoneNumber) { },
/**
* @event
* @param {string} callType
* @param {string} phoneNumber
* @type void
*/
onCallEvent: function(callType, phoneNumber) { },
/**
* @event
* @param {array} callRecordsFound
* @type void
*/
onCallRecordsFound: function(callRecordsFound) { }
};
/**
* Feature URI: http://jil.org/jil/api/1.1/callrecord
*
* @namespace Widget.Telephony.CallRecord
* @version 1.2.2
*/
Widget.Telephony.CallRecord = {
/** @type string */
callRecordAddress: "",
/** @type string */
callRecordId: "",
/** @type string */
callRecordName: "",
/** @type string */
callRecordType: "",
/** @type number */
durationSeconds: new Number(),
/** @type Date */
startTime: new Date()
};
/**
* Feature URI: http://jil.org/jil/api/1.1.1/callrecordtypes
*
* @namespace Widget.Telephony.CallRecordTypes
* @version 1.2.2
*/
Widget.Telephony.CallRecordTypes = {
/** @type string */
MISSED: "",
/** @type string */
OUTGOING: "",
/** @type string */
RECEIVED: ""
};